WorldViewClient version 2.0¶
Overview¶
One of the main features of ROSVITA is the WorldView. The WorldView is the place in which the current configration is visualized. But it not only visualize the current state of the work cell it is also the place where we can jog the robot, save specific poses or robot joint configurations and add collision objects which should be considered when new trajectories are planned and executed.
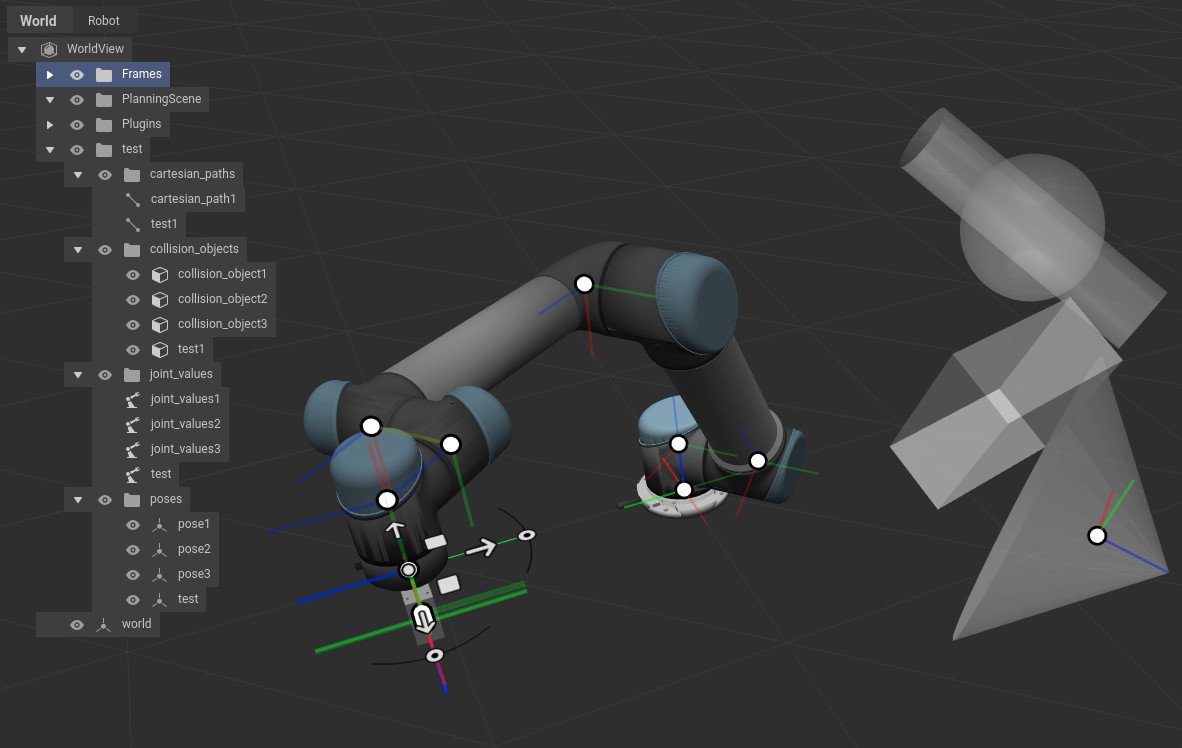
ROSVITA WorldView example¶
To get access to the stored entities in WorldView or to add new or manipulate
existing onces the xamla_motion.v2.WorldViewClient
class can be used.
At the moment following functionalities are provided:
Add and remove folders from WorldView
Add, get, update, query and remove poses, joint values/configurations, cartesian paths and collision objects.
The version 2.0 is an update to the now deprecated implementation xamla_motion.WorldViewClient
.
In version 2.0 a lot of API changes are made to improve the usability.
Major changes are:
add and update world view elements is now done with help of the add methods
update methods are removed
all methods now use a full path instead of a split path (folder_path and element_name)
add_folder default behavior not raise a exception if folder already exists
remove_element default behavior not raise exception if element not exists
Example:
from xamla_motion.v2.world_view_client import WorldViewClient
from xamla_motion.data_types import JointValues, JointSet, Pose, CartesianPath
from xamla_motion.data_types import CollisionObject, CollisionPrimitive
from pyquaternion import Quaternion
def main():
# create entities which should be added to and manipulated in world view
joint_set1 = JointSet(['Joint1', 'Joint2', 'Joint3'])
joint_values1 = JointValues(joint_set1, [1.0, 2.0, 3.0])
joint_set2 = JointSet(['Joint1', 'Joint2'])
joint_values2 = JointValues(joint_set2, [19.0, 20.0])
joint_set3 = JointSet(['Joint1', 'Joint4'])
joint_values3 = JointValues(joint_set3, [100.0, 30.0])
t1 = [0.502522, 0.2580, 0.3670]
q1 = Quaternion(w=0.304389, x=0.5272, y=0.68704, z=0.39666)
t2 = [0.23795, 0.46845, 0.44505]
q2 = Quaternion(w=0.212097, x=0.470916, y=0.720915, z=0.462096)
t3 = [0.6089578, 0.3406782, 0.208865]
q3 = Quaternion(w=0.231852, x=0.33222, y=0.746109, z=0.528387)
pose1 = Pose(t1, q1, 'world')
pose2 = Pose(t2, q2, 'world')
pose3 = Pose(t3, q3, 'world')
cartesian_path1 = CartesianPath([pose1, pose2, pose3])
sphere = CollisionPrimitive.create_sphere(0.1, pose2)
cylinder = CollisionPrimitive.create_cylinder(0.4, 0.05, pose2)
box = CollisionPrimitive.create_box(0.2, 0.2, 0.1, pose1)
plane = CollisionPrimitive.create_plane(1.0, 1.0, 1.0, 1.0, pose3)
cone = CollisionPrimitive.create_cone(0.2, 0.2, pose3)
collision_object1 = CollisionObject([box])
collision_object2 = CollisionObject([cylinder])
collision_object3 = CollisionObject([plane, cone])
# create a instance of WorldViewClient to get access to rosvita world view
world_view_client = WorldViewClient()
print('---------------- add folder --------------')
# add folder test at WorldView root/test
world_view_client.add_folder('test/joint_values')
world_view_client.add_folder('test/poses')
world_view_client.add_folder('test/cartesian_paths')
world_view_client.add_folder('test/collision_objects')
print('---------------- add joint values --------------')
world_view_client.add_joint_values(
'test/joint_values/joint_values1',
joint_values1
)
world_view_client.add_joint_values(
'test/joint_values/test',
joint_values2
)
print('---------------- update joint values --------------')
world_view_client.add_joint_values(
'test/joint_values/joint_values1',
joint_values2
)
print('---------------- get joint values --------------')
get_value = world_view_client.get_joint_values(
'test/joint_values/joint_values1'
)
print('joint_values1 is: ' + str(get_value))
print('---------------- query joint values --------------')
# query all values which start with t under test/joint_values
queried_values = world_view_client.query_joint_values(
'test/joint_values',
't'
)
for v in queried_values:
print(str(v))
print('---------------- add poses --------------')
world_view_client.add_pose(
'test/poses/pose1',
pose1
)
world_view_client.add_pose(
'test/poses/test',
pose3
)
print('---------------- update pose --------------')
world_view_client.add_pose(
'test/poses/pose1',
pose2
)
print('---------------- get pose --------------')
get_value = world_view_client.get_pose(
'test/poses/pose1'
)
print('pose1 is: ' + str(get_value))
print('---------------- query poses --------------')
# query all poses which start with t under test/pose
queried_values = world_view_client.query_poses('test/poses', 't')
for v in queried_values:
print(str(v))
print('---------------- add cartesian path --------------')
world_view_client.add_cartesian_path(
'test/cartesian_paths/cartesian_path1',
cartesian_path1
)
world_view_client.add_cartesian_path(
'test/cartesian_paths/test',
cartesian_path1
)
print('---------------- update cartesian path --------------')
world_view_client.add_cartesian_path(
'test/cartesian_paths/cartesian_path1',
cartesian_path1
)
print('---------------- get cartesian path --------------')
get_value = world_view_client.get_cartesian_path(
'test/cartesian_paths/cartesian_path1'
)
print('cartesian_path1 is: ' + str(get_value))
print('---------------- query cartesian paths --------------')
# query all cartesian_paths which start with t under test/cartesian_path
queried_values = world_view_client.query_cartesian_paths(
'test/cartesian_paths',
't'
)
for v in queried_values:
print(str(v))
print('---------------- add collision object --------------')
world_view_client.add_collision_object(
'test/collision_objects/collision_object1',
collision_object1
)
world_view_client.add_collision_object(
'test/collision_objects/test',
collision_object1
)
print('---------------- update collision object --------------')
world_view_client.add_collision_object(
'test/collision_objects/collision_object1',
collision_object2
)
print('---------------- get collision object --------------')
get_value = world_view_client.get_collision_object(
'test/collision_objects/collision_object1'
)
print('collision_object1 is: ' + str(get_value))
print('---------------- query collision object --------------')
# query all collision_objects which start with t under test/collision_object
queried_values = world_view_client.query_collision_objects(
'test/collision_objects',
't'
)
for v in queried_values:
print(str(v))
input('Press enter to clean up')
print('----------------- remove folder test ---------------')
world_view_client.remove_element('test', '')
if __name__ == '__main__':
main()
WorldViewClient¶
-
class
xamla_motion.v2.
WorldViewClient
[source]¶ Client to interact and manipulate the Rosvita WorldView
With help of this client class it is possible to add, get, query and remove different kinds of elements to or from the Rosvita world view.
-
query_cartesian_pahts
()¶ Query all existing castesian paths under folder_path which start with prefix
-
query_cartesian_pahts
() Query all existing castesian paths under folder_path which start with prefix
Initialize WorldViewClient
- Returns
- Return type
Instance of WorldViewClient
- Raises
ServiceError – If connection to world view services could not be established
-
add_cartesian_path
(element_path, cartesian_path, transient=False, update_if_exists=True)[source] Add / store cartesian path element in world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Path in world view tree where the new cartesian path element should be created or update a existing one at this location
cartesian_path (CartesianPath) – Instance of cartesian path which is added / stored
transient (bool) – If True the added cartesian path is only valid for this session and will not be saved in a rosvita project context
update_if_exists (bool (default True)) – If True update cartesian path if it exists instead of raising an exception
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to add cartesian path due to wrong path or element already exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it If cartesian_path is not of type CartesianPath
-
add_collision_object
(element_path, collision_object, transient=False, update_if_exists=True)[source] Add / store collision object element in world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Path in world view tree where the new collision object element should be created or update a existing one at this location
collision_object (CollisionObject) – Instance of collision object which is added or used for update
transient (bool) – If True the added collision object is only valid for this session and will not be saved in a rosvita project context
update_if_exists (bool (default True)) – If True update cartesian path if it exists instead of raising an exception
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to add collision object due to wrong path or element already exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it If collision_object is not of type CollisionObject
-
add_folder
(folder_path, raise_exception_if_exists=False)[source]¶ Add a folder to world view tree
- Parameters
folder_path (Union[str, pathlib.Path]) – Folder path in worldview tree
raise_exception_if_exists (bool (default False)) – If True raise ArgumentError is folder already exists als no exception is raised and folder existing folder presists.
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to add folder input path not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-
add_joint_values
(element_path, joint_values, transient=False, update_if_exists=True)[source] Add / update joint values element in world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Path in world view tree where the new joint values element should be created or update a existing one at this location
joint_values (JointValues) – Contains new joint values
transient (bool (default False)) – If True the added joint values are only valid for this session and will not be saved in a rosvita project context
update_if_exists (bool (default True)) – If True update the values if exists instead of raising an exception
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to add joint values due to wrong path or element already exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it If joint_values is not of type JointValues
-
add_pose
(element_path, pose, transient=False, update_if_exists=True)[source] Add / store pose element in world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Path in world view tree where the new pose element should be created or update a existing one at this location
pose (Pose) – Instance of pose which is added / stored
transient (bool) – If True the added pose is only valid for this session and will not be saved in a rosvita project context
update_if_exists (bool (default True)) – If True update pose if it exists instead of raising an exception
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to add pose due to wrong path or element already exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it If pose is not of type Pose
-
get_cartesian_path
(element_path)[source] Get cartesian path element from world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Full path of requested element in worldview tree
- Returns
cartesian_path – Instance of CartesianPath with the values which are defined in the requested cartesian path element
- Return type
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to get cartesian path due to wrong path or element not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-
get_collision_object
(element_path)[source] Get collision object element from world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Full path of requested element in worldview tree
- Returns
collision_object – Instance of CollisionObject with the values which are defined in the requested collision object element
- Return type
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to get collision object due to wrong path or element not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-
get_joint_values
(element_path)[source] Get joint values element from world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Full path of requested element in worldview tree
- Returns
joint_values – Instance of JointValues with the values which are defined in the requested joint values element
- Return type
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to get joint values due to wrong path or element not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-
get_pose
(element_path)[source] Get pose element from world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Full path of requested element in worldview tree
- Returns
pose – Instance of Pose with the values which are defined in the requested pose element
- Return type
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to get pose due to wrong path or element not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-
query_cartesian_paths
(folder_path, prefix='', recursive=False)[source]¶ Query all existing cartesian path elements under folder_path which start with prefix
- Parameters
- Returns
result – List of CartesianPath in alphanumeric order see world view
- Return type
List[CartesianPath]
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to query cartesian path due to not existing path
TypeError – If folder_path is not of type str
-
query_collision_objects
(folder_path, prefix='', recursive=False)[source]¶ Query all existing collision objet elements under folder_path which start with prefix
- Parameters
- Returns
result – List of CollisionObject in alphanumeric order see world view
- Return type
List[CollisionObject]
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to query collision object due to not existing path
TypeError – If folder_path is not of type str
-
query_joint_values
(folder_path, prefix='', recursive=False)[source] Query all existing joint values elements under folder_path which start with prefix
- Parameters
- Returns
result – List of JointValues in alphanumeric order see world view
- Return type
List[JointValues]
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to query joint values due to not existing path
TypeError – If folder_path is not of type str
-
query_poses
(folder_path, prefix='', recursive=False)[source] Query all existing pose elements under folder_path which start with prefix
-
remove_element
(element_path, raise_exception_if_not_exists=False)[source]¶ Remove existing element from world view tree
- Parameters
element_path (Union[str, pathlib.Path]) – Folder path in worldview tree
raise_exception_if_not_exists (bool default(False)) – If True raise a ArgumentError if element which should be removed not exists
- Raises
ServiceError – If necessary service is not available
ArgumentError – If it was not possible to update collision object due to element not exists
TypeError – If element_path is not of type pathlib.Path and can not be converted to it
-